Go through the basic JavaScript skills before interview --- Prototype and prototype chain
Questions:
a. How to check if an object is an array?
b. Create a simple jQuery manually.
c. How do you understand the relationship between prototype and class?
Skills:
1. Type check - instanceof:
class People {
constructor(name) {
this.name = name
}
eat() {
console.log(`${this.name} eat something`)
}
}
class Student extends People {
constructor(name, number) {
super(name)
this.number = number
}
sayHi() {
console.log(`name ${this.name} number ${this.number}`)
}
}
const lux = new Student('Lux', 100)
lux instanceof Student // true
lux instanceof People // true, People is parent of Student
lux instanceof Object // true, Object is parent of all classes
[] instanceof Array // true
[] instanceof Object // true
{} instanceof Object // true
2. Prototype
// in fact, class is a function, so I consider class as a syntax sugar
typeof People // 'function'
typeof Student // 'function'
// implicit prototype and explicit prototype
console.log( lux.__proto__ )
console.log( Student.prototype )
console.log(lux.__proto__ === Student.prototype ) // true
3. Prototype chain and instanceof
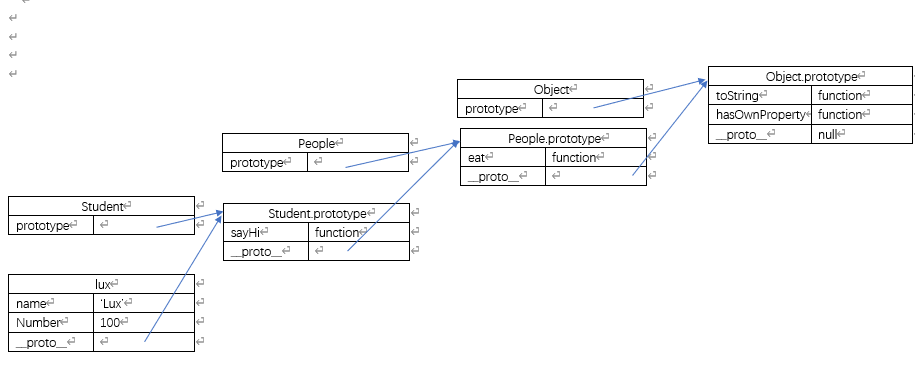
a. Every class has an explicit prototype prototype
;
b. Every instance has an implicit prototype __proto__
;
c. The implicit prototype of instance points to the explicit prototype of its class;
d. When require the properties or functions, JS will not search from the __proto__
until can't get it from the properties and functions which belong to this instance.
e. instanceof
will follow the prototype chain and search the name of each prototype, and will return true
once find one.
4. Write a simple jQuery
class jQuery {
constructor(selector) {
const result = document.querySelectorAll(selector)
const length = result.length
for (let i = 0; i < length; i++) {
this[i] = result[i]
}
this.length = length
this.selector = selector
}
get(index) {
return this[index]
}
each(fn) {
for (let i = 0; i < this.length; i++) {
const elem = this[i]
fn(elem)
}
}
on(type, fn) {
return this.each(elem => {
elem.addEventListener(type, fn, false)
})
}
// extend more DOM APIs
}
// use prototype to extend jQuery
jQuery.prototype.dialog = function (info) {
alert(info)
}
// use class inheritance to extend jQuery
class myJQuery extends jQuery {
constructor(selector) {
super(selector)
}
// extend own functions
addClass(className) {
}
style(data) {
}
}
// const $p = new jQuery('p')
// $p.get(1)
// $p.each((elem) => console.log(elem.nodeName))
// $p.on('click', () => alert('clicked'))